Problem 2463 Minimum Total Distance Traveled
Table of Contents
Problem Statement
Link - Problem 2463
Question
There are some robots and factories on the X-axis. You are given an integer array robot
where robot[i]
is the position of the ith
robot. You are also given a 2D integer array factory
where factory[j] = [positionj, limitj]
indicates that positionj
is the position of the jth
factory and that the jth
factory can repair at most limitj
robots.
The positions of each robot are unique. The positions of each factory are also unique. Note that a robot can be in the same position as a factory initially.
All the robots are initially broken; they keep moving in one direction. The direction could be the negative or the positive direction of the X-axis. When a robot reaches a factory that did not reach its limit, the factory repairs the robot, and it stops moving.
At any moment, you can set the initial direction of moving for some robot. Your target is to minimize the total distance traveled by all the robots.
Return the minimum total distance traveled by all the robots. The test cases are generated such that all the robots can be repaired.
Note that
- All robots move at the same speed.
- If two robots move in the same direction, they will never collide.
- If two robots move in opposite directions and they meet at some point, they do not collide. They cross each other.
- If a robot passes by a factory that reached its limits, it crosses it as if it does not exist.
- If the robot moved from a position
x
to a positiony
, the distance it moved is|y - x|
.
Example 1:
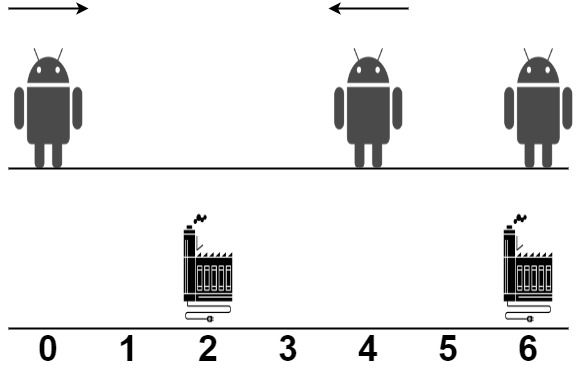
Input: robot = [0,4,6], factory = [[2,2],[6,2]] Output: 4 Explanation: As shown in the figure: - The first robot at position 0 moves in the positive direction. It will be repaired at the first factory. - The second robot at position 4 moves in the negative direction. It will be repaired at the first factory. - The third robot at position 6 will be repaired at the second factory. It does not need to move. The limit of the first factory is 2, and it fixed 2 robots. The limit of the second factory is 2, and it fixed 1 robot. The total distance is |2 - 0| + |2 - 4| + |6 - 6| = 4. It can be shown that we cannot achieve a better total distance than 4.
Example 2:
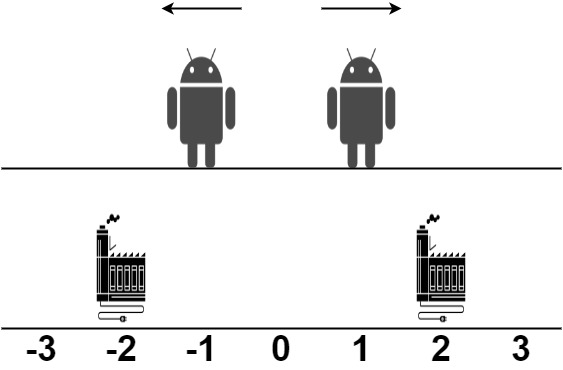
Input: robot = [1,-1], factory = [[-2,1],[2,1]] Output: 2 Explanation: As shown in the figure: - The first robot at position 1 moves in the positive direction. It will be repaired at the second factory. - The second robot at position -1 moves in the negative direction. It will be repaired at the first factory. The limit of the first factory is 1, and it fixed 1 robot. The limit of the second factory is 1, and it fixed 1 robot. The total distance is |2 - 1| + |(-2) - (-1)| = 2. It can be shown that we cannot achieve a better total distance than 2.
Constraints:
1 <= robot.length, factory.length <= 100
factory[j].length == 2
-109 <= robot[i], positionj <= 109
0 <= limitj <= robot.length
- The input will be generated such that it is always possible to repair every robot.
Solution
class Solution {
public:
long long minimumTotalDistance(vector<int>& robot, vector<vector<int>>& factory) {
sort(robot.begin(), robot.end());
sort(factory.begin(), factory.end());
vector<int> factoryPositions;
for (auto& f : factory)
for (int i = 0; i < f[1]; i++)
factoryPositions.push_back(f[0]);
int robotCount = robot.size(), factoryCount = factoryPositions.size();
vector<vector<long long>> memo(robotCount, vector<long long>(factoryCount, -1));
return calculateMinDistance(0, 0, robot, factoryPositions, memo);
}
long long calculateMinDistance(int robotIdx, int factoryIdx, vector<int>& robot, vector<int>& factoryPositions, vector<vector<long long>>& memo) {
if (robotIdx == robot.size())
return 0;
if (factoryIdx == factoryPositions.size())
return 1e12;
if (memo[robotIdx][factoryIdx] != -1)
return memo[robotIdx][factoryIdx];
long long assign = abs(robot[robotIdx] - factoryPositions[factoryIdx]) + calculateMinDistance(robotIdx + 1, factoryIdx + 1, robot, factoryPositions, memo);
long long skip = calculateMinDistance(robotIdx, factoryIdx + 1, robot, factoryPositions, memo);
return memo[robotIdx][factoryIdx] = min(assign, skip);
}
};
Complexity Analysis
| Algorithm | Time Complexity | Space Complexity |
| ------------------------------------ | --------------- | ---------------- |
| Dynamic Programming with Memoization | O(n m) | O(nm) |
Explanation
Intial Thoughts
To approach this problem, consider the positions of the robots and factories on the X-axis. Think of the robots as needing to move to the nearest available factory that has not reached its repair limit. The goal is to minimize the total distance traveled. Key points include: sorting the robots and factories by position to efficiently process them, using dynamic programming to store and reuse the results of subproblems, and considering the trade-offs between assigning a robot to the current factory versus skipping it and moving on to the next one.
Intuitive Analysis
Intuitively, the problem can be solved by visualizing the movement of the robots as a series of decisions about which factory to move towards, given the constraints on the number of robots each factory can repair. The analysis involves breaking down the problem into smaller subproblems, such as deciding whether to assign a robot to the current factory or to skip it, and using the results of these subproblems to inform the overall solution. Key points include: the importance of sorting the input to enable efficient processing, the use of memoization to avoid redundant calculations, and the need to balance the competing demands of minimizing distance traveled with the constraints on factory capacity.
1. Intuition
- The problem can be approached by first sorting the robot and factory positions to simplify the problem space
- We need to consider the optimal assignment of robots to factories to minimize the total distance traveled
- The key insight is to use dynamic programming to explore all possible assignments and find the optimal one
- The problem can be broken down into smaller sub-problems by considering each robot and factory position individually
- The optimal solution will involve a trade-off between assigning a robot to a nearby factory versus skipping it and assigning it to a farther factory
- The use of memoization is crucial to avoid redundant calculations and improve the efficiency of the dynamic programming approach
- The base cases for the dynamic programming approach are when all robots have been assigned or when all factories have been considered
2. Implementation
- The
minimumTotalDistance
function initializes the sorting of robot and factory positions and sets up the dynamic programming tablememo
- The
calculateMinDistance
function is a recursive function that explores all possible assignments of robots to factories using dynamic programming - The
assign
variable calculates the distance traveled if the current robot is assigned to the current factory, and theskip
variable calculates the distance traveled if the current robot is not assigned to the current factory - The
memo
table is used to store the results of sub-problems to avoid redundant calculations - The
factoryPositions
vector is used to store the positions of the factories, and therobot
vector is used to store the positions of the robots - The
calculateMinDistance
function returns the minimum distance traveled by considering all possible assignments of the current robot to the current factory or skipping it - The
minimumTotalDistance
function returns the minimum total distance traveled by all robots by calling thecalculateMinDistance
function with the initial indices
Complexity Analysis
Time Complexity:
- The time complexity is determined by the nested loops in the
calculateMinDistance
function and the sorting of therobot
andfactory
vectors. ThecalculateMinDistance
function has a recursive structure with memoization, resulting in a time complexity of O(n * m), where n and m are the sizes of therobot
andfactoryPositions
vectors, respectively. - The dominant operations are the recursive calls to
calculateMinDistance
and the comparisons in themin
function. Each cell in the memoization table is filled once, resulting in a total of n * m operations. - The Big O classification of O(n _ m) is justified because the number of operations grows linearly with the product of the sizes of the input vectors. The sorting operations at the beginning have a time complexity of O(n log n) and O(m log m), but they are dominated by the O(n _ m) complexity of the dynamic programming algorithm.
Space Complexity:
- The space complexity is determined by the size of the memoization table
memo
, which has a size of n * m, where n and m are the sizes of therobot
andfactoryPositions
vectors, respectively. - The impact of the data structure is significant, as the memoization table is the largest data structure used in the algorithm. The space complexity is directly proportional to the product of the sizes of the input vectors.
- The space complexity of O(n _ m) is justified because the algorithm needs to store the results of subproblems in the memoization table to avoid redundant computations. The input vectors
robot
andfactory
also require O(n) and O(m) space, respectively, but these are dominated by the O(n _ m) space required by the memoization table.
Footnote
This question is rated as Hard difficulty.
Hints
Sort robots and factories by their positions.
After sorting, notice that each factory should repair some subsegment of robots.
Find the minimum total distance to repair first i robots with first j factories.
Similar Questions:
Title | URL | Difficulty |
---|---|---|
Capacity To Ship Packages Within D Days | https://leetcode.com/problems/capacity-to-ship-packages-within-d-days | Medium |
Number of Ways to Earn Points | https://leetcode.com/problems/number-of-ways-to-earn-points | Hard |